Particle effects¶
GDX Particle Editor¶
Kõige lihtsamalt saab kvaliteetseid efekte oma LibGDX mängule luua GDX Particle Editor-ist, kus on juba olema 13 valmisefekti ning samuti võimalus ise luua.
![]() splash¶ |
![]() smoke¶ |
![]() explosion¶ |
![]() rain¶ |
![]() fireworks¶ |
![]() sparks¶ |
![]() glitch¶ |
![]() thruster¶ |
![]() flame¶ |
![]() demolition¶ |
![]() infinity¶ |
![]() smoke¶ |
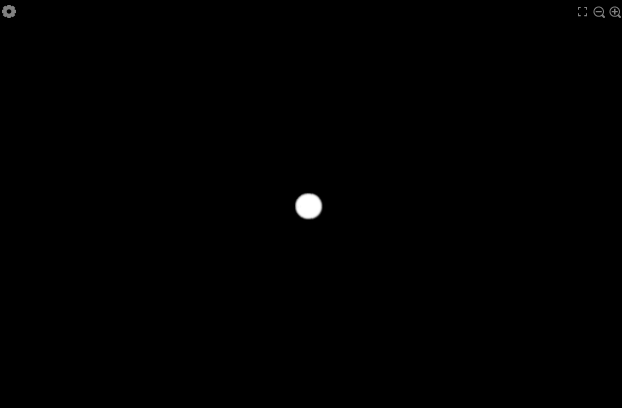
trail¶
Esmalt on vaja alla laadida rakenduse JAR-fail https://github.com/libgdx/gdx-particle-editor/releases Kui see tehtud, valime paigutusrežiimi.
![]() Wizard layout¶ |
![]() Classic layout¶ |
Režiimi saab muuta maja ikooni kaudu, mis asub ekraani all paremas nurgas.

Vaikimisi on ekraanil tuleleegi efekt. Teised ette tehtud efektid on leitavad Effect Emitters
aknast Template
nupu alt.
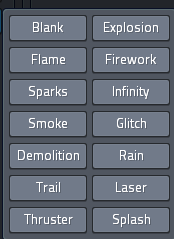
Omaenda efektide tegemiseks on põhjalik juhend LibGDX Particle Editor
dokumentatsioonist https://github.com/libgdx/gdx-particle-editor/wiki/In%E2%80%90Depth-Guide/
Kõik need efektid põhinevad PNG-failidest, mida saab alla laadida järgmisest kaustast https://github.com/libgdx/gdx-particle-editor/files/13857004/particle-images.zip
Oma PNG-failide kasutamiseks on vaja Emitter Properties
akna Image
sektsioonis fail üles-laadida Add
nupu kaudu.
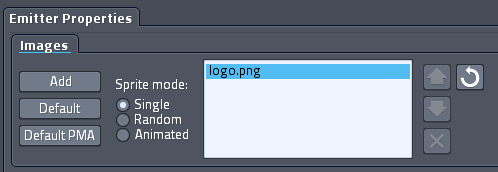
Koodis particle effects kasutamine¶
Seejärel loome Game
klassi laiendava klassi, et ei peaks TextureAtlas
't mitu korda looma ning deklareerime kohe PNG-failid, millele hiljem viitame P-failide viimasel real.
Vaikimisi oli nende PNG-failidel article.png
ja particle-cloud.png
, ma vahetasin enda projektis need flame.png
ning smoke.png
vastu.
Selles klassis määrame ekraani setScreen
meetodi abil.
public class MyGdxGame extends Game {
static public TextureAtlas textureAtlas;
@Override
public void create () {
textureAtlas = new TextureAtlas();
textureAtlas.addRegion("smoke",new TextureRegion(new Texture("smoke.png")));
textureAtlas.addRegion("flame",new TextureRegion(new Texture("flame.png")));
this.setScreen(new GameScreen(this));
}
@Override
public void render () {
super.render();
}
}
Screen
-i laiendavas klassis loome kaks Actor
-objekti, et siis hiljem mõlema objekti enda klassis neile efektid külge panna. BucketActor
-i paneme liikuma üles-alla, et demonstreerida, kuidas efekt Actor
-i külge jääb.
public class GameScreen implements Screen {
private final Stage stage;
public TextureAtlas textureAtlas;
public GameScreen(Game game) {
textureAtlas = new TextureAtlas();
stage = new Stage(new ScreenViewport());
Soda bucket = new Soda();
bucket.setX(Gdx.graphics.getWidth() / 12);
stage.addActor(bucket);
Bucket drop = new Bucket();
drop.setPosition(Gdx.graphics.getWidth() * 3 / 5, Gdx.graphics.getHeight() / 5);
drop.addAction(Actions.repeat(-1, Actions.sequence(Actions.moveTo(Gdx.graphics.getWidth() * 3 / 5, Gdx.graphics.getHeight() * 3 / 5, 2, Interpolation.sine), Actions.moveTo(Gdx.graphics.getWidth() * 3 / 5, Gdx.graphics.getHeight() / 5, 2, Interpolation.sine))));
stage.addActor(drop);
}
@Override
public void render(float delta) {
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
stage.act();
stage.draw();
}
}
Järgmisena peame Actor
-id looma. Teeme neile oma kausta.
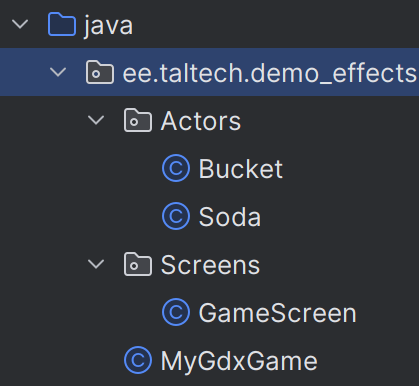
SodaActor
-i külge lisame suitsuefekti.
public class Soda extends Image {
ParticleEffect effect;
public Soda() {
super(new Texture("soda.png"));
effect = new ParticleEffect();
effect.load(Gdx.files.internal("smoke.p"), MyGdxGame.textureAtlas);
effect.start();
effect.setPosition(this.getWidth() + this.getX(), this.getHeight() + this.getY());
}
@Override
public void draw(Batch batch, float parentAlpha) {
super.draw(batch, parentAlpha);
effect.draw(batch);
}
@Override
public void act(float delta) {
super.act(delta);
effect.update(delta);
}
@Override
public void setX(float x) {
super.setX(x);
effect.setPosition(this.getWidth() + this.getX(), this.getHeight() + this.getY());
}
}
smoke.p
faili viimane rida peab viitama pildile, mille määrasime Game
klassi laiendavas klassis.
Untitled
- Delay -
active: false
- Duration -
lowMin: 3000.0
lowMax: 3000.0
- Count -
min: 0
max: 200
- Emission -
lowMin: 0.0
lowMax: 0.0
highMin: 5.0
highMax: 5.0
relative: false
scalingCount: 1
scaling0: 1.0
timelineCount: 1
timeline0: 0.0
- Life -
lowMin: 0.0
lowMax: 0.0
highMin: 6000.0
highMax: 6000.0
relative: false
scalingCount: 1
scaling0: 1.0
timelineCount: 1
timeline0: 0.0
independent: false
- Life Offset -
active: false
independent: false
- X Offset -
active: false
- Y Offset -
active: false
- Spawn Shape -
shape: point
- Spawn Width -
lowMin: 0.0
lowMax: 0.0
highMin: 0.0
highMax: 0.0
relative: false
scalingCount: 1
scaling0: 1.0
timelineCount: 1
timeline0: 0.0
- Spawn Height -
lowMin: 0.0
lowMax: 0.0
highMin: 0.0
highMax: 0.0
relative: false
scalingCount: 1
scaling0: 1.0
timelineCount: 1
timeline0: 0.0
- X Scale -
lowMin: 40.0
lowMax: 40.0
highMin: 80.0
highMax: 80.0
relative: true
scalingCount: 2
scaling0: 0.0
scaling1: 1.0
timelineCount: 2
timeline0: 0.0
timeline1: 1.0
- Y Scale -
active: false
- Velocity -
active: true
lowMin: 0.0
lowMax: 0.0
highMin: 50.0
highMax: 50.0
relative: false
scalingCount: 1
scaling0: 1.0
timelineCount: 1
timeline0: 0.0
- Angle -
active: true
lowMin: 0.0
lowMax: 0.0
highMin: 75.0
highMax: 105.0
relative: false
scalingCount: 1
scaling0: 1.0
timelineCount: 1
timeline0: 0.0
- Rotation -
active: true
lowMin: 0.0
lowMax: 360.0
highMin: -100.0
highMax: 100.0
relative: true
scalingCount: 2
scaling0: 0.0
scaling1: 1.0
timelineCount: 2
timeline0: 0.0
timeline1: 1.0
- Wind -
active: false
- Gravity -
active: false
- Tint -
colorsCount: 3
colors0: 0.5764706
colors1: 0.5764706
colors2: 0.5764706
timelineCount: 1
timeline0: 0.0
- Transparency -
lowMin: 0.0
lowMax: 0.0
highMin: 1.0
highMax: 1.0
relative: false
scalingCount: 4
scaling0: 0.0
scaling1: 1.0
scaling2: 1.0
scaling3: 0.0
timelineCount: 4
timeline0: 0.0
timeline1: 0.21527798
timeline2: 0.805556
timeline3: 1.0
- Options -
attached: false
continuous: true
aligned: false
additive: false
behind: false
premultipliedAlpha: false
spriteMode: single
- Image Paths -
smoke.png
BucketActor
külge lisame tuleleegi efekti. fire.p
faili viimane rida peab samuti viitama Game
klassi laiendavas klassis deklareeritud pildile.
public class Bucket extends Image {
ParticleEffect effect;
public Bucket() {
super(new Texture("bucket.png"));
effect = new ParticleEffect();
effect.load(Gdx.files.internal("fire.p"), MyGdxGame.textureAtlas);
effect.start();
effect.setPosition(this.getWidth() / 2 + this.getX(), this.getHeight() / 2 + this.getY());
}
@Override
public void draw(Batch batch, float parentAlpha) {
super.draw(batch, parentAlpha);
effect.draw(batch);
}
@Override
public void act(float delta) {
super.act(delta);
effect.setPosition(this.getWidth() / 2 + this.getX(), this.getHeight() / 2 + this.getY());
effect.update(delta);
}
}
Tulemus:
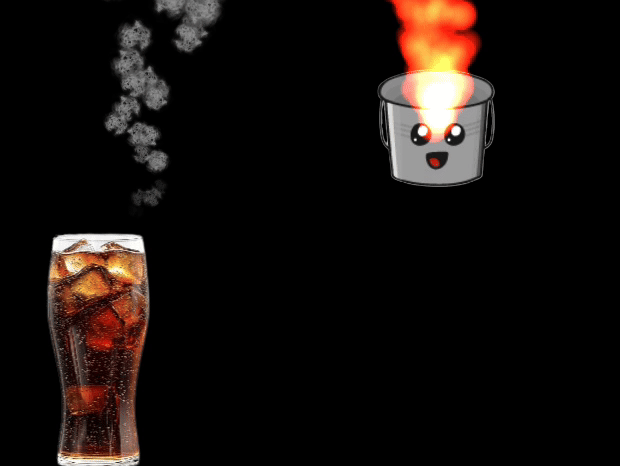
Kõik mainitud lingid¶
GDX Particle Editor JAR-fail https://github.com/libgdx/gdx-particle-editor/releases
GDX Particle Editor dokumentatsioon https://github.com/libgdx/gdx-particle-editor/wiki/In%E2%80%90Depth-Guide/
Kõik GDX Particle Editor PNG-failid https://github.com/libgdx/gdx-particle-editor/files/13857004/particle-images.zip